API Reference
The Paykickstart API allows for a wide variety of interactions with the Paykickstart Platform using an application.
The API endpoint is located here: https://app.paykickstart.com/api
- All API requests should use the above URL as their base url.
- All API requests must be made over HTTPS. Calls made over plain HTTP will fail. API requests without authentication will also fail.
With the exception of the Instant Payment Notification system which uses a different validation method, every api call must include an auth_token field, which is located in your Platform Settings, under “API Key”. This key is used to verify the vendor’s account and respective API access permissions.
Responses from the Paykickstart API are returned in JSON format, unless otherwise stipulated.
Instant Payment Notification
IPN POST
IPN Validation Function
PHP
<?php
function is_valid_ipn($data, $secret_key) {
// Hash received
$ipnHash = $data['hash'];
// Unset encrypted keys
unset($data['hash'], $data['verification_code']);
// Trim and ommit empty/null values from the data
$data = array_filter(array_map('trim', $data));
foreach ($data as $key => $item) {
$data[$key] = iconv("UTF-8","ISO-8859-1//IGNORE",$item);
}
// Alphabetically sort IPN parameters by their key. This ensures
// the params are in the same order as when Paykickstart
// generated the verification code, in order to prevent
// hash key invalidation due to POST parameter order.
ksort($data, SORT_STRING);
// Implode all the values into a string, delimited by "|"
// Generate the hash using the imploded string and secret key
$hash = hash_hmac( 'sha1', implode("|", $data), $secret_key );
return $hash == $ipnHash;
}
JS
var Crypto={sha1_hmac:function(r,t){function e(t){const e=[];for(let r=0;r<t.length;r++){var o=t.charCodeAt(r);o<128?e.push(o):(o<2048?e.push(192|o>>6):(o<65536?e.push(224|o>>12):(e.push(240|o>>18),e.push(128|o>>12&63)),e.push(128|o>>6&63)),e.push(128|63&o))}return e}function o(t){let e="";for(let r=0;r<t.length;r++)e+=String.fromCharCode(t[r]);return e}let h=e(t);if(64<h.length){const f=Crypto.sha1(o(h),!0);h=[];for(let r=0;r<f.length;r++)h.push(f.charCodeAt(r))}const a=Array(64).fill(0);for(let r=0;r<h.length;r++)a[r]=h[r];const n=Array(64).fill(54),s=Array(64).fill(92);for(let r=0;r<64;r++)n[r]^=a[r],s[r]^=a[r];r=e(r),r=o(n)+o(r),r=Crypto.sha1(r,!0),r=o(s)+r;return Crypto.sha1(r)},sha1:function(r,t){function e(r,t){return r<<t|r>>>32-t}function o(r,t){for(var e,o="",h=7;0<=h;h--)e=r>>>4*h&15,o+=t?String.fromCharCode(e):e.toString(16);return o}for(var h,a,n,s,f,c,u,p,C,l=Array(80),i=1732584193,A=4023233417,d=2562383102,g=271733878,m=3285377520,y=r.length,b=[],v=0;v<y-3;v+=4)a=r.charCodeAt(v)<<24|r.charCodeAt(v+1)<<16|r.charCodeAt(v+2)<<8|r.charCodeAt(v+3),b.push(a);switch(y%4){case 0:v=2147483648;break;case 1:v=r.charCodeAt(y-1)<<24|8388608;break;case 2:v=r.charCodeAt(y-2)<<24|r.charCodeAt(y-1)<<16|32768;break;case 3:v=r.charCodeAt(y-3)<<24|r.charCodeAt(y-2)<<16|r.charCodeAt(y-1)<<8|128}for(b.push(v);b.length%16!=14;)b.push(0);for(b.push(y>>>29),b.push(y<<3&4294967295),h=0;h<b.length;h+=16){for(v=0;v<16;v++)l[v]=b[h+v];for(v=16;v<=79;v++)l[v]=e(l[v-3]^l[v-8]^l[v-14]^l[v-16],1);for(v=0,n=i,s=A,f=d,c=g,u=m;v<=19;v++)temp=e(n,5)+(s&f|~s&c)+u+l[v]+1518500249&4294967295,u=c,c=f,f=e(s,30),s=n,n=temp;for(v=20;v<=39;v++)temp=e(n,5)+(s^f^c)+u+l[v]+1859775393&4294967295,u=c,c=f,f=e(s,30),s=n,n=temp;for(v=40;v<=59;v++)temp=e(n,5)+(s&f|s&c|f&c)+u+l[v]+2400959708&4294967295,u=c,c=f,f=e(s,30),s=n,n=temp;for(v=60;v<=79;v++)temp=e(n,5)+(s^f^c)+u+l[v]+3395469782&4294967295,u=c,c=f,f=e(s,30),s=n,n=temp;i=i+n&4294967295,A=A+s&4294967295,d=d+f&4294967295,g=g+c&4294967295,m=m+u&4294967295}if(p=(o(i)+o(A)+o(d)+o(g)+o(m)).toLowerCase(),!t)return p;for(C="";p.length;)C+=String.fromCharCode(parseInt(p.substr(0,2),16)),p=p.substr(2);return C}};
/**
*
* @param { {} } $data
* @param {string} $secret_key
* @return {boolean}
*/
function is_valid_ipn($data, $secret_key)
{
let $ipnHash = $data.hash,
completedData = [];
delete $data.hash;
delete $data.verification_code;
let data = Object.keys($data)
.sort()
.reduce(function (acc, key) {
acc[key] = $data[key];
return acc;
}, {});
for (let key in data) {
if (data.hasOwnProperty(key)) {
let value = data[key];
if (value !== '' && value !== '0' && value !== 0 && value !== null) {
completedData.push(String(value));
}
}
}
let $hash = Crypto.sha1_hmac(completedData.join("|"), $secret_key);
return $hash === $ipnHash;
}
PYTHON
import hmac
import hashlib
def is_valid_ipn(data, secret):
"""
:param data: json object with input data. i.e. data from post query
:param secret: string
"""
ipn_hash = data['hash']
completed_data = []
del data['hash'], data['verification_code']
for attr, value in sorted(data.items()):
if value != '' and value != '0' and value != 0 and value != None:
completed_data.append(cleanString(value))
h = hmac.new(secret.encode(), "|".join(map(str, completed_data)).encode(), hashlib.sha1)
return h.hexdigest() == ipn_hash
def cleanString(input):
if (isinstance(input, str)):
return ''.join([i if ord(i) < 128 else '' for i in input])
return input
PayKickstart’s Instant Payment Notification (IPN) is a message service that automatically notifies vendors of events related to PayKickstart transactions. Vendors can use it to automate back-office and administrative functions, including automatically creating users on apps, providing customers with their login credentials via email etc.
ARGUMENTS
event |
Stipulates the type of transaction event which has occurred. Each IPN that’s fired is fired based on a specific event, so it’s possible that a single transaction can fire multiple IPNs, particularly in the case of subscriptions. Please note that these IPN events may not be fired in any particular order. The event types are:
|
mode |
Indicates whether the transaction was executed in “live” mode or “test” mode. The parameter’s possible value are:
|
payment_processor |
Indicates the payment gateway used to create the transaction. Supported payment gateways include:
|
amount |
The transaction amount |
buyer_ip |
The transaction’s buyer’s details |
buyer_first_name |
|
buyer_last_name |
|
buyer_email |
|
vendor_first_name |
The transaction’s Paykickstart vendor’s details |
vendor_last_name |
|
vendor_email |
|
billing_address_1 |
The transaction’s buyer’s billing details |
billing_address_2 |
|
billing_city |
|
billing_state |
|
billing_zip |
|
billing_country |
|
shipping_address_1 |
The transaction’s buyer’s shipping details |
shipping_address_2 |
|
shipping_city |
|
shipping_state |
|
shipping_zip |
|
shipping_country |
|
transaction_id |
The unique Paykickstart transaction ID |
invoice_id |
The unique Paykickstart purchase ID |
old_invoice_id |
The original Paykickstart purchase ID of the old subscription (only included in the subscription-changed event) |
tracking_id |
The transaction’s Paykickstart affiliate’s tracking link id |
transaction_time |
The time when the transaction was generated, in UNIX timestamp format. |
product_id |
The transaction’s product details |
product_name |
|
campaign_id |
The transaction’s campaign details |
campaign_name |
|
funnel_id |
The transaction’s funnel ID |
funnel_name |
|
affiliate_first_name |
The transaction’s Paykickstart affiliate’s details and the amount paid to the affiliate for this transaction. Both the commission value and percentage amounts are indicated. |
affiliate_last_name |
|
affiliate_email |
|
affiliate_commission_amount |
|
affiliate_commission_percent |
|
ref_affiliate_first_name |
The transaction’s Paykickstart 2nd tier affiliate’s details and the amount paid to that affiliate for this transaction. Both the commission value and percentage amounts are indicated. |
ref_affiliate_last_name |
|
ref_affiliate_email |
|
ref_affiliate_commission_amount |
|
ref_affiliate_commission_percent |
|
update_billing_url |
* Only available in the subscription-payment-failed IPN event. Returns the URL where the customer may update their payment details for the subscription in which their payment failed. |
next_billing_date |
* Only available in the sales IPN event, and only if applicable (i.e. not populated in the case of 1-time transactions). Returns the unix timestamp of when the subscription will next charge (in GMT0 timezone). |
custom_{field} |
Field names prefixed with “custom_” in the IPN POST are custom fields which you may set in the checkout page URL. For example, if your Paykickstart checkout page URL is https://app.paykickstart.com/checkout/123, you can add additional information to the URL in the form of GET variables like this: https://app.paykickstart.com/checkout/123?var1=123&var2=email@user.com Continuing with the example above, we’d pass these custom variables back to you in the IPN POST, like this: custom_var1 = 123 custom_var2 = ’email@user.com’ |
licenses |
Returns any licenses linked to the transaction, either as an array if more than 1 license, or a string if only 1 license. |
hash |
This is a hashed security key that can be used to validate that the IPN POST received is in fact valid / verified. You should generate a hash using the function provided (see IPN Validation Function on the left) and compare it against the hash within the POST. If they match, the IPN is verified. The secret key variable required by the IPN validation function is located in your campaign settings (see screenshot below). |
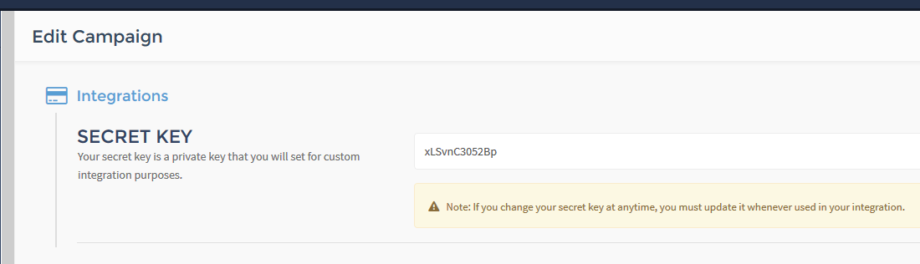
Affiliate IPN POST
PayKickstart’s Affiliate Instant Payment Notification (IPN) is a message service that automatically notifies affiliates of events related to PayKickstart transactions.
amount |
The transaction amount |
buyer_first_name |
The transaction’s buyer’s details. These fields may not reflect if the vendor has chosen to disable them. |
buyer_last_name |
|
tracking_id |
The transaction’s Paykickstart affiliate’s tracking link id |
transaction_time |
The time when the transaction was generated, in UNIX timestamp format. |
product_name |
The transaction’s product details |
campaign_name |
The transaction’s campaign details |
affiliate_first_name |
The transaction’s Paykickstart affiliate’s details and the amount paid to the affiliate for this transaction. |
affiliate_last_name |
|
affiliate_email |
|
affiliate_commission_amount |
|
Customer Portal
Login Customer
POST
/billing-customer
Login Customer
Allows the user to fetch the login token of a customer for a customer portal iframe. This is useful for vendors who want to embed the customer portal into a membership area where the vendor already knows the customer’s email address and doesn’t want to require the customer to have to use the email verification login system on the portal (auto-login).
USAGE:
Once you’ve received the login token in the API response, you need to modify the iframe source URL and include a new parameter called “secret”. The value of that parameter should be the token you received in the response.
Example URI
Arguments
auth_token |
|
The email address of the customer. |
Affiliates
The following API calls deal with retrieving affiliate data and marketing materials
Get All Affiliates
POST
/affiliates/all
Get All Affiliates
Returns all the affiliate’s details for a specified campaign.
Example URI
Arguments
auth_token |
|
campaign_id |
The affiliate’s campaign’s id. |
Get Affiliate
POST
/affiliate
Get Affiliate
Returns the affiliate’s details and links.
Example URI
Arguments
auth_token |
|
campaign_id |
The affiliate’s campaign’s id. |
affiliate |
The affiliate’s email address or ID. |
Licensing
PayKickstart’s licensing system is a service which creates and manages licenses on a per-product basis for vendors who activate the service.The service is activated by enabling “Licensing” in the Product Details section when editing a product. The “License Usage” field indicates the number of licenses which will be issued each time the product is purchased.
The issued licenses are given to the customer on Paykickstart’s default “thank you” page and on Paykickstart’s Order Lookup page. They are also indicated in the IPN service. The link to Paykickstart’s Order Lookup page is included by default to the buyer’s sales receipt email.
Get License Data
POST
/licenses/data
Get License Data
This GET request returns license information for a specific license key
Example URI
Arguments
auth_token |
|
license_key |
|
Get License Status
POST
/licenses/status
Get License Status
This GET request returns license status information for a specific license key
Example URI
Arguments
auth_token |
|
license_key |
|
Activate License
POST
/licenses/activate
Activate License
This POST request activates the license for a specific license key / GUID combination
Example URI
Arguments
auth_token |
|
license_key |
|
guid |
The GUID is usually a 128-bit integer number used to identify unique resources, but for the purpose of activating a license on Paykickstart it can be any unique hash or “identifier” to identify where the license has been activated, such as a unique hardware identifier, or a unique URL. This prevents the license from being activated in multiple environments at once (1 license per use). |
Clear License "De-Register"
POST
/licenses/clear
Clear License "De-Register"
This POST request “clears” / de-registers the license from its specified GUID, allowing it to be activated again for a new (or the same) GUID
Example URI
Arguments
auth_token |
|
license_key |
|
Enable License
POST
/licenses/enable
Enable License
This POST request enables the license for being activated and verified
Example URI
Arguments
auth_token |
|
license_key |
|
Disable License
POST
/licenses/disable
Disable License
This POST request disables the license from being activated or verified
Example URI
Arguments
auth_token |
|
license_key |
|
Reissue License
POST
/licenses/reissue
Reissue License
This POST request reissue the license
NOTE: The license key must first be "cleared" / de-registered before it can be reissued.
Example URI
Arguments
auth_token |
|
license_key |
|
New Purchase
PayKickstart’s New Purchase API call is a highly flexible system designed to allow you as the vendor complete flexibility in terms of creating and managing new purchases. Using the API, you’re able to override your products’ default price settings, or even create a new charge without needing a client’s credit card information (reference transaction).
Step 1 (Tokenization)
The Paykickstart API supports accepting payment processor tokens in order to effect payment as opposed to raw card data. It is therefore a TWO-PART or THREE-PART (if customer authorization is required) system. The first part involves creating tokens directly from your checkout page using javascript provided by your payment processor, and the second part involves sending those tokens to the Paykickstart API endpoint.
We have created an example page which you can access to view the javascript required for each of your processors in each of your campaigns, as well as a copy-and-paste javascript you can use on your checkout pages to simplify the process.
In order to access this, please login to your Paykickstart vendor account, then go to this URL: https://app.paykickstart.com/test-code-v2/pci. The page which loads will list all your current campaigns and their associated processor integrations:
Click “go” next to the campaign you are integrating with using the API. On the following page, you’ll find tabs at the top for your supported payment types (credit card, SEPA, ACH etc.) and an “all” option. Clicking on any of those tabs will provide you with a sample form and javascript, as well as the done for you, copy-and-paste javascript sample at the top of the page.
The done-for-you PK tokenize javascript MUST BE PLACED BELOW THE PURCHASE FORM ELEMENT in the DOM structure. It accepts the following GET variables:
ccNum |
|
ccExpireMonth |
|
ccExpireYear |
|
ccCSV |
|
ccBtn |
|
ppBtn |
|
form |
|
ccExpireYear |
|
wt_account_holder_name |
|
sepa_email |
|
iban_number |
|
sepaBtn |
|
route_number |
|
account_number |
|
achBtn |
|
ccExpireYear |
|
billing_address_1 |
|
billing_address_2 |
|
billing_city |
|
billing_state |
|
billing_zip |
|
billing_country |
|
You may choose to NOT use that done for you PK tokenisation script if your form is a bit more complex, in which case the example javascript provided may be copied to your own form and modified as required:
You can also fill in the form field provided on the page and click “click me” to see an example output of the working javascript:
Using the generated token, you can submit an API call (see below) to process the transaction.
Step 2 (New Purchase Request)
POST
/purchase
Step 2 (New Purchase Request)
Example URI
auth_token |
|
product |
|
plan |
|
affiliate_id |
|
first_name |
|
last_name |
|
|
|
ref_purchase |
|
test_mode |
|
price |
|
quantity |
|
coupon_code |
|
split_selected |
|
total_installments |
|
price_per_installment |
|
is_recurring |
|
recurring_freq |
|
recurring_freq_type |
|
cycles |
|
has_trial |
|
trial_amount |
|
trial_days |
|
buyer_tax_number |
|
buyer_tax_name |
|
capture_billing_address |
|
billing_address_1 |
|
billing_address_2 |
|
billing_city |
|
billing_state |
|
billing_zip |
|
billing_country |
|
billing_phone_code |
|
billing_phone_number |
|
capture_shipping_address |
|
shipping_address_1 |
|
shipping_address_2 |
|
shipping_city |
|
shipping_state |
|
shipping_zip |
|
shipping_country |
|
shipping_phone_code |
|
shipping_phone_number |
|
tos-read |
|
ccNum |
|
ccExpireYear |
|
ccExpireMonth |
|
custom_field[<FIELDNAME>] |
|
Below outlines the token fields required in the API call depending on your payment processor:
Stripe Credit Card:
If you have NOT enabled Strong Customer Authentication (SCA) for your Stripe integration:
stripeToken |
|
If you HAVE enabled Strong Customer Authentication (SCA) for your Stripe integration:
stripePm |
|
Stripe SEPA:
stripeSourceId |
|
sepa |
|
wt-type |
|
Stripe ACH:
stripeSourceId |
|
route_number |
|
account_number |
|
stripeBAToken |
|
wt-type |
|
Braintree Credit Card:
pay_method_nonce |
|
Authorize.net:
dataDescriptor |
|
dataValue |
|
Easy Pay Direct:
epdSaleToken |
|
epdCustomerToken |
|
PayPal / Braintree PayPal:
paypal_ba_id |
|
Connect:
payment_method_token |
|
Step 3 (Verification)
If you are using Stripe and you have SCA enabled, you may need to request authorization from your customer to process the transaction. Paykickstart will inform you if this is the case in the response from step 2 by returning a status of “requires_action”.
In this case, you will need to load a page which includes Stripe’s javascript that’ll open a modal asking your customer to authorize the transaction – please see the example provided on https://app.paykickstart.com/test-code-v2/pci.
Once the customer authorizes the transaction, you will need to submit another API call to Paykickstart as follows:
POST
https://app.paykickstart.com/api/confirm-payment
Arguments
auth_token |
|
invoice_id |
|
stripePi |
|
NOTE: you MUST submit the above request to the endpoint indicated above even if authorization fails or is not completed by the customer!
Payments
The following API calls offer checkout and payment-related API functions.
Get Purchase
POST
/purchase/get
Get Purchase
Returns the purchase details.
Example URI
Arguments
auth_token |
|
id |
The purchase’s id. |
Get Transaction
POST
/transaction/get
Get Transaction
Returns the transaction details.
Example URI
Arguments
auth_token |
|
id |
The transaction’s id. |
Update Credit Card
POST
/purchase/update-cc
Update Credit Card
This POST request updates a customer’s credit card information for a specified purchase. Please note that we DO NOT store your customer’s credit card details in Paykickstart. Paykickstart uses frontend tokenisation technology to protect you and your customers from passing payment information insecurely, and all information supplied via this API call is directly relayed to the linked payment processor where the payment profile is updated.
Example URI
Arguments
auth_token |
|
invoice_id |
|
PaymentProcessorTokens |
The payment processor token is required. Please refer to the new purchase documentation on how to generate tokens and which parameters to set. |
ccNum |
The last 4 digits of the customer’s credit card number. |
ExpireMonth |
The 2-digit expiry month of the customer’s credit card. |
ExpireYear |
The 4-digit expiry year of the customer’s credit card. |
Get Transactions (last 100)
POST
/transactions
Get Transactions (last 100)
Fetches the last 100 transactions (or 100 transactions up to the created_at date) for the associated account.
Example URI
Arguments
auth_token |
|
created_at |
The end date up to when to fetch the transactions in UNIX timestamp format. |
affiliate_id |
Fetch all affiliate transactions. |
Refund Transaction
POST
/transaction/refund
Refund Transaction
Example URI
Arguments
auth_token |
|
transaction_id |
The transaction’s id. |
Subscription
The subscriptions methods allow you to update a subscription’s status and set the next charge date, or to cancel the subscription.
Cancel Subscription
POST
/subscriptions/cancel
Cancel Subscription
This POST request cancels an active subscription
Example URI
Arguments
auth_token |
|
invoice_id |
|
cancel_at |
Optional field to set a specific cancellation date (unix timestamp). |
fire_event |
Toggle whether or not Paykickstart should fire all the subscription cancellation events. This field is not required and default is 1 (true). |
charge_overage |
Toggle whether or not Paykickstart should calculate and charge any overage fees due during cancellation. This field is not required and default is 0 (false). |
Reactivate Subscription
POST
/subscriptions/re-activate
Reactivate Subscription
This POST request reactivates a canceled subscription
Example URI
Arguments
auth_token |
|
invoice_id |
|
date |
Set the next billing date (Unix timestamp).This field is required. |
Change The Next Subscription Billing Date
POST
/subscriptions/change_next_date
Change The Next Subscription Billing Date
This POST request changes the next billing date of an active subscription
NOTE: The Subscription must be "Active".
Example URI
Arguments
auth_token |
|
invoice_id |
|
date |
Set the next billing date (Unix timestamp).This field is required. |
Up/Downgrade Subscription
POST
/subscriptions/change
Up/Downgrade Subscription
This POST request changes a subscription by activating the new subscription first, then cancelling the current subscription.
Example URI
Arguments
auth_token |
|
invoice_id |
|
product_id |
The new product with which subscription should now be associated. |
charge_date |
The next time the customer will be charged for the subscription. This field is optional; if not provided then the next charge date will remain unchanged. |
first_charge |
This is the pro-rata amount to charge/credit immediately. To add a credit instead of a charge, pass a NEGATIVE value in this parameter. This field is not required; if not provided the system will automatically calculate the amount to charge or to credit. |
allowed_units |
If usage is enabled, this parameter allows you to provide the usage limit for the pro-rata period. This field is not required; if not provided the system will automatically calculate it. |
coupon_code |
Apply a specific coupon code to the subscription. |
Subscriptions Usage
POST
/subscriptions/usage
Subscriptions Usage
This POST request allows you to add usage to a subscription
Example URI
Arguments
auth_token |
|
invoice_id |
|
units |
Set the number of usage units to attribute to this subscription for the current subscription period. This field can be negative if you wish to “credit” units to a specific subscription for the current subscriptino period. |
notes |
Add a comment/reason for the usage / credit. This field is not required. |
Subscriptions Add Credit
POST
/subscriptions/credit/add
Subscriptions Add Credit
This POST request allows you to add credit to a subscription
Example URI
Arguments
auth_token |
|
subscription_id |
The unique Paykickstart subscription ID |
amount |
Set the amount of credit to this subscription. This field can be negative. |
note |
Add a comment/reason for the credit. This field is not required. |
Set Subscription Payment Method
POST
/subscriptions/set-pm
Set Subscription Payment Method
Example URI
auth_token |
|
invoice_id |
|
type |
|
If your "type" is "cc", then you need send the following data inside request:
ccNum |
|
ccExpireMonth |
|
ccExpireYear |
|
If your gateway one of braintree, paypalvault then next field is required:
pay_method_nonce |
|
If your gateway is stripe then next field is required:
payment_method |
|
source_id |
|
If your gateway is epd then next field is required:
payment_token |
|
If your gateway is authnet then next field is required:
dataDescriptor |
|
dataValue |
|
If your gateway is connect then next field is required:
payment_method_token |
|
country |
|
postal_code |
|
Set Subscription Upgrade/Downgrade terms
POST
/subscriptions/upgrade/wording
Get Subscription Upgrade/Downgrade terms
Example URI
auth_token |
|
invoice_id |
|
product_id |
The new product with which subscription should now be associated. |
first_charge |
This is the pro-rata amount to charge/credit immediately. To add a credit instead of a charge, pass a NEGATIVE value in this parameter. This field is not required; if not provided the system will automatically calculate the amount to charge or to credit. |
charge_date |
The next time the customer will be charged for the subscription. This field is optional; if not provided then the next charge date will remain unchanged. |
allowed_units |
If usage is enabled, this parameter allows you to provide the usage limit for the pro-rata period. This field is not required; if not provided the system will automatically calculate it. |
Update subscription's customer details
POST
/update-address
Update subscription customer new details
Example URI
auth_token |
|
invoice_id |
|
billing_address_1 |
|
billing_address_2 |
|
billing_city |
|
billing_state |
|
billing_zip |
|
billing_country |
|
shipping_address_1 |
|
shipping_address_2 |
|
shipping_city |
|
shipping_state |
|
shipping_zip |
|
shipping_country |
|
shipping_same_as_billing |
|
buyer_first_name |
|
buyer_last_name |
|
buyer_email |
|
Coupons
This section describes all the available coupon API calls
Create Coupon
POST
/coupons
Create Coupon
Creates a new coupon.
Example URI
Arguments
auth_token |
|
coupon_name |
The display name for your coupon. |
coupon_code |
The Unique coupon code for your coupon. |
coupon_rate |
The value of your coupon. |
coupon_type |
The type of value – accepted arguments are: “amount” and “percentage” |
coupon_apply_to |
Determines if the coupon should be applied to first charge (excludes split pay), first charge, rebills and/or split payments. This is an array parameter where one or more of the below arguments are accepted:
|
coupon_start_date |
The start date of when the coupon is active. Correct format '12/31/2022 00:00:00' |
coupon_end_date |
The end date of when the coupon is active. Correct format '12/31/2023 00:00:00' |
coupon_max_redemption |
The maximum number of times a coupon may be redeemed |
plan_ids |
An array of plan ids which the coupon should be applied to |
product_id |
The campaign id which the coupon should be applied to |
affiliate_id |
The affiliate ID which should be applied to the coupon |
affiliate_id_tier_2 |
The affiliate ID which should be applied to the coupon |
coupon_rebills_number |
Coupon Rebills Number. Applies only when coupon_apply_to contains 3. |
Check Coupon Status
POST
/coupon/status
Check Coupon Status
Check status of the coupon.
Example URI
Calculate Coupon
POST
/coupon/calculate
Calculate Coupon
Calculate data for coupon
Example URI
Leads
This section describes all the available leads API calls
Confirm Lead
POST
/leads/confirm
Confirm Lead
Allows the user to confirm an unconfirmed lead.
Example URI
Arguments
auth_token |
|
lead_id |
The lead’s id. |
lead_campaign_id |
The Lead Campaign’s id. |
Invoices
This section describes all the available invoice API calls
Get Invoice
POST
/invoice
Get Invoice
Allows the user to get the link to invoice pdf file.
Example URI
Arguments
auth_token |
|
transaction_id |
The transaction PK id. |